559. N 叉树的最大深度
559. N 叉树的最大深度
🟢 🔖 树
深度优先搜索
广度优先搜索
🔗 力扣
LeetCode
题目
Given a n-ary tree, find its maximum depth.
The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.
Nary-Tree input serialization is represented in their level order traversal, each group of children is separated by the null value (See examples).
Example 1:
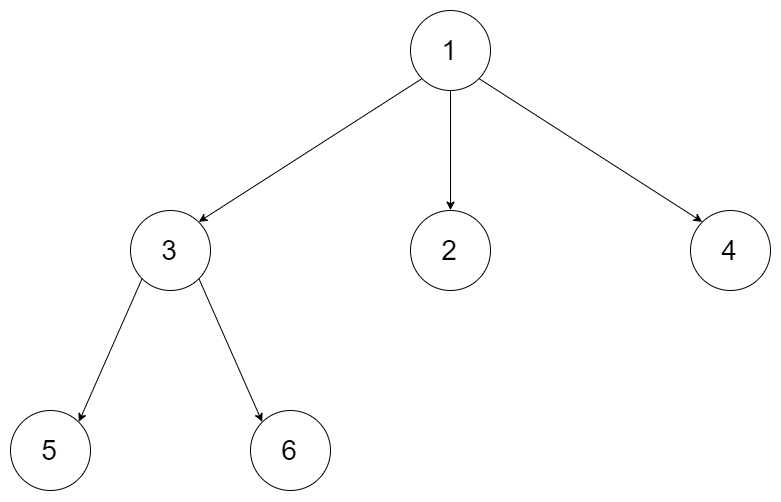
Input: root = [1,null,3,2,4,null,5,6]
Output: 3
Example 2:
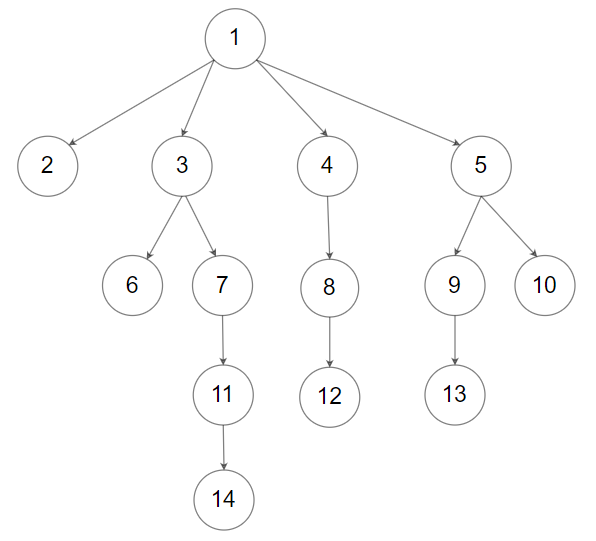
Input: root = [1,null,2,3,4,5,null,null,6,7,null,8,null,9,10,null,null,11,null,12,null,13,null,null,14]
Output: 5
Constraints:
- The total number of nodes is in the range
[0, 10^4]
. - The depth of the n-ary tree is less than or equal to
1000
.
题目大意
给定一个 N 叉树,找到其最大深度。
最大深度是指从根节点到最远叶子节点的最长路径上的节点总数。
N 叉树输入按层序遍历序列化表示,每组子节点由空值分隔(请参见示例)。
解题思路
思路和 第 104 题 二叉树的最大深度 一样。
思路一:递归
只需求出根节点的每个子树的最大高度,取出其中的最大值再加一即为根节点的最大高度。
思路二:回溯
深度优先搜索(DFS)一颗 N 叉树,在 DFS 中,递归返回的时候,我们需要进行回溯(backtrack)。depth
变量用来记录当前节点的深度,每次进入一个子节点时,depth
增加,每次返回到父节点时,depth
需要减少。
代码
/**
* @param {Node|null} root
* @return {number}
*/
var maxDepth = function (root) {
if (!root) return 0;
let max = 0;
for (let i of root.children) {
max = Math.max(max, maxDepth(i));
}
return max + 1;
};
/**
* @param {Node|null} root
* @return {number}
*/
var maxDepth = function (root) {
let depth = 0;
let res = 0;
const traverse = (root) => {
if (!root) return null;
depth++;
res = Math.max(depth, res);
for (let i of root.children) {
traverse(i);
}
depth--;
};
traverse(root);
return res;
};
相关题目
题号 | 标题 | 题解 | 标签 | 难度 |
---|---|---|---|---|
104 | 二叉树的最大深度 | [✓] | 树 深度优先搜索 广度优先搜索 1+ | |
2039 | 网络空闲的时刻 | 广度优先搜索 图 数组 | ||
3249 | 统计好节点的数目 | 树 深度优先搜索 |