1528. 重新排列字符串
1528. 重新排列字符串
题目
You are given a string s
and an integer array indices
of the same length. The string s
will be shuffled such that the character at the ith
position moves to indices[i]
in the shuffled string.
Return the shuffled string.
Example 1:
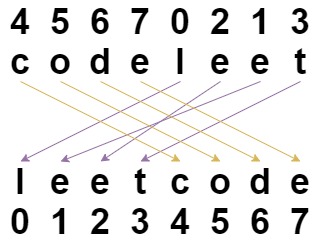
Input: s = "codeleet", indices = [4,5,6,7,0,2,1,3]
Output: "leetcode"
Explanation: As shown, "codeleet" becomes "leetcode" after shuffling.
Example 2:
Input: s = "abc", indices = [0,1,2]
Output: "abc"
Explanation: After shuffling, each character remains in its position.
Constraints:
s.length == indices.length == n
1 <= n <= 100
s
consists of only lowercase English letters.0 <= indices[i] < n
- All values of
indices
are unique.
题目大意
给你一个字符串 s
和一个 长度相同 的整数数组 indices
。
请你重新排列字符串 s
,其中第 i
个字符需要移动到 indices[i]
指示的位置。
返回重新排列后的字符串。
示例 1:
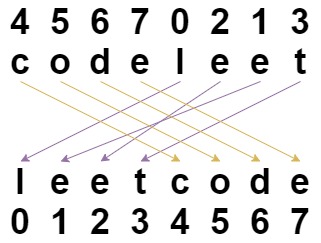
输入: s = "codeleet", indices = [4,5,6,7,0,2,1,3]
输出: "leetcode"
解释: 如图所示,"codeleet" 重新排列后变为 "leetcode" 。
示例 2:
输入: s = "abc", indices = [0,1,2]
输出: "abc"
解释: 重新排列后,每个字符都还留在原来的位置上。
提示:
s.length == indices.length == n
1 <= n <= 100
s
仅包含小写英文字母0 <= indices[i] < n
indices
的所有的值都是 唯一 的
解题思路
创建一个与字符串
s
长度相同的数组res
用于存储重新排列的字符。遍历字符串和
indices
数组,对于字符串s
中的每个字符以及对应的indices[i]
,将字符放到结果数组的正确位置:res[indices[i]] = s[i]
使用
join
方法将数组res
转换为一个字符串。
复杂度分析
- 时间复杂度:
O(n)
,其中n
是字符串的长度,需要遍历字符串一次。 - 空间复杂度:
O(n)
,使用了一个长度为n
的结果数组res
。
代码
/**
* @param {string} s
* @param {number[]} indices
* @return {string}
*/
var restoreString = function (s, indices) {
let res = new Array(s.length); // 初始化结果数组
for (let i = 0; i < s.length; i++) {
res[indices[i]] = s[i]; // 根据索引重新排列字符
}
return res.join(''); // 转换为字符串并返回
};