1812. 判断国际象棋棋盘中一个格子的颜色
1812. 判断国际象棋棋盘中一个格子的颜色
题目
You are given coordinates
, a string that represents the coordinates of a square of the chessboard. Below is a chessboard for your reference.
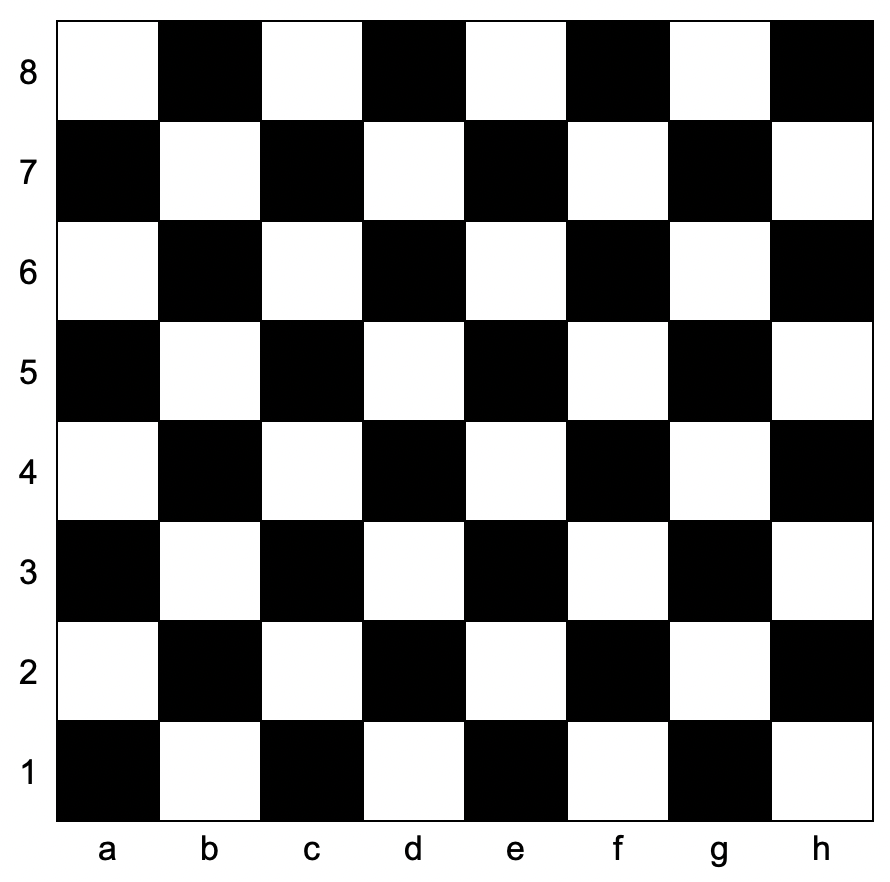
Return true
if the square is white, andfalse
if the square is black.
The coordinate will always represent a valid chessboard square. The coordinate will always have the letter first, and the number second.
Example 1:
Input: coordinates = "a1"
Output: false
Explanation: From the chessboard above, the square with coordinates "a1" is black, so return false.
Example 2:
Input: coordinates = "h3"
Output: true
Explanation: From the chessboard above, the square with coordinates "h3" is white, so return true.
Example 3:
Input: coordinates = "c7"
Output: false
Constraints:
coordinates.length == 2
'a' <= coordinates[0] <= 'h'
'1' <= coordinates[1] <= '8'
题目大意
给你一个坐标 coordinates
,它是一个字符串,表示国际象棋棋盘中一个格子的坐标。下图是国际象棋棋盘示意图。

如果所给格子的颜色是白色,请你返回 true
,如果是黑色,请返回 false
。
给定坐标一定代表国际象棋棋盘上一个存在的格子。坐标第一个字符是字母,第二个字符是数字。
示例 1:
输入: coordinates = "a1"
输出: false
解释: 如上图棋盘所示,"a1" 坐标的格子是黑色的,所以返回 false 。
示例 2:
输入: coordinates = "h3"
输出: true
解释: 如上图棋盘所示,"h3" 坐标的格子是白色的,所以返回 true 。
示例 3:
输入: coordinates = "c7"
输出: false
提示:
coordinates.length == 2
'a' <= coordinates[0] <= 'h'
'1' <= coordinates[1] <= '8'
解题思路
坐标系统:棋盘上的坐标由字母和数字组成,例如
a1
,h8
。- 字母表示列(
a
到h
),即从左到右的 8 列。 - 数字表示行(
1
到8
),即从下到上的 8 行。
- 字母表示列(
格子颜色的规律:
- 对于标准的棋盘,颜色是交替的,黑白格子交替出现。
- 如果
(列号 + 行号) % 2 == 0
,则是黑色格子,否则是白色格子。- 例如,
a1
是黑色的,b1
是白色的,a2
是白色的,b2
是黑色的,依此类推。
- 例如,
列转换:
- 首先需要将字母表示的列(例如
a
、b
)转换为数字。a
映射到 1,b
映射到 2,以此类推。 - 可以通过
charCodeAt
来获取字母的 ASCII 值,再减去'a'
的 ASCII 值并加上 1,得到列号。
- 首先需要将字母表示的列(例如
判断颜色:
- 根据列号和行号的和
(列号 + 行号) % 2
来判断格子的颜色。 - 如果
(列号 + 行号) % 2 == 1
,则是白色格子。
- 根据列号和行号的和
复杂度分析
时间复杂度:
O(1)
,只需要进行常数时间的字符和数字操作。空间复杂度:
O(1)
,只使用了常数空间来存储列号和行号。
代码
/**
* @param {string} coordinates
* @return {boolean}
*/
var squareIsWhite = function (coordinates) {
// 获取列号
const col = coordinates[0].charCodeAt() - 'a'.charCodeAt() + 1;
// 获取行号
const row = Number(coordinates[1]);
// 判断是否是白色格子
return (col + row) % 2 == 1;
};
相关题目
题号 | 标题 | 题解 | 标签 | 难度 | 力扣 |
---|---|---|---|---|---|
3274 | 检查棋盘方格颜色是否相同 | 数学 字符串 | 🟢 | 🀄️ 🔗 |