100. Same Tree
100. Same Tree
🟢 🔖 树
深度优先搜索
广度优先搜索
二叉树
🔗 LeetCode
题目
Given the roots of two binary trees p
and q
, write a function to check if they are the same or not.
Two binary trees are considered the same if they are structurally identical, and the nodes have the same value.
Example 1:
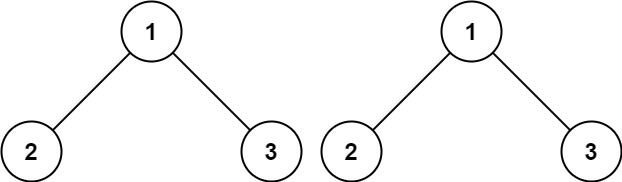
Input: p = [1,2,3], q = [1,2,3]
Output: true
Example 2:
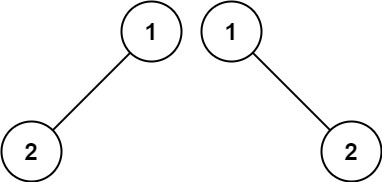
Input: p = [1,2], q = [1,null,2]
Output: false
Example 3:
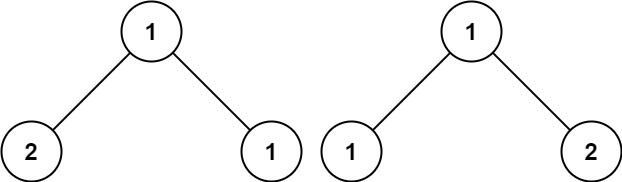
Input: p = [1,2,1], q = [1,1,2]
Output: false
Constraints:
- The number of nodes in both trees is in the range
[0, 100]
. -10^4 <= Node.val <= 10^4
题目大意
这一题要求判断两颗树是否是完全相等的。
如果两个树在结构上相同,并且节点具有相同的值,则认为它们是相同的。
解题思路
递归判断即可。
代码
/**
* @param {TreeNode} p
* @param {TreeNode} q
* @return {boolean}
*/
var isSameTree = function (p, q) {
if (p == null && q == null) return true;
else if (p != null && q != null) {
if (p.val != q.val) {
return false;
}
return isSameTree(p.left, q.left) && isSameTree(p.right, q.right);
}
return false;
};