2194. Excel 表中某个范围内的单元格
2194. Excel 表中某个范围内的单元格
题目
A cell (r, c)
of an excel sheet is represented as a string "<col><row>"
where:
<col>
denotes the column numberc
of the cell. It is represented by alphabetical letters.- For example, the
1st
column is denoted by'A'
, the2nd
by'B'
, the3rd
by'C'
, and so on.
- For example, the
<row>
is the row numberr
of the cell. Therth
row is represented by the integerr
.
You are given a string s
in the format "<col1><row1>:<col2><row2>"
, where <col1>
represents the column c1
, <row1>
represents the row r1
, <col2>
represents the column c2
, and <row2>
represents the row r2
, such that r1 <= r2
and c1 <= c2
.
Return the list of cells (x, y)
such that r1 <= x <= r2
and c1 <= y <= c2
. The cells should be represented as strings in the format mentioned above and be sorted in non-decreasing order first by columns and then by rows.
Example 1:
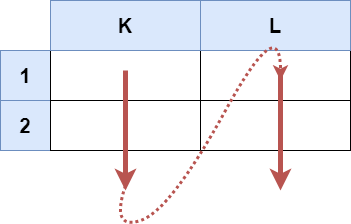
Input: s = "K1:L2"
Output: ["K1","K2","L1","L2"]
Explanation:
The above diagram shows the cells which should be present in the list.
The red arrows denote the order in which the cells should be presented.
Example 2:

Input: s = "A1:F1"
Output: ["A1","B1","C1","D1","E1","F1"]
Explanation:
The above diagram shows the cells which should be present in the list.
The red arrow denotes the order in which the cells should be presented.
Constraints:
s.length == 5
'A' <= s[0] <= s[3] <= 'Z'
'1' <= s[1] <= s[4] <= '9'
s
consists of uppercase English letters, digits and':'
.
题目大意
Excel 表中的一个单元格 (r, c)
会以字符串 "<col><row>"
的形式进行表示,其中:
<col>
即单元格的列号c
。用英文字母表中的 字母 标识。- 例如,第
1
列用'A'
表示,第2
列用'B'
表示,第3
列用'C'
表示,以此类推。
- 例如,第
<row>
即单元格的行号r
。第r
行就用 整数r
标识。
给你一个格式为 "<col1><row1>:<col2><row2>"
的字符串 s
,其中 <col1>
表示 c1
列,<row1>
表示 r1
行,<col2>
表示 c2
列,<row2>
表示 r2
行,并满足 r1 <= r2
且 c1 <= c2
。
找出所有满足 r1 <= x <= r2
且 c1 <= y <= c2
的单元格,并以列表形式返回。单元格应该按前面描述的格式用 字符串 表示,并以 非递减 顺序排列(先按列排,再按行排)。
示例 1:
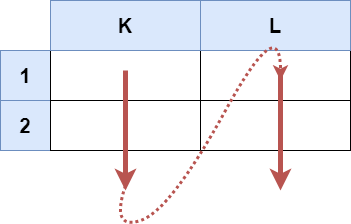
输入: s = "K1:L2"
输出:["K1","K2","L1","L2"]
解释:
上图显示了列表中应该出现的单元格。
红色箭头指示单元格的出现顺序。
示例 2:

输入: s = "A1:F1"
输出:["A1","B1","C1","D1","E1","F1"]
解释:
上图显示了列表中应该出现的单元格。
红色箭头指示单元格的出现顺序。
提示:
s.length == 5
'A' <= s[0] <= s[3] <= 'Z'
'1' <= s[1] <= s[4] <= '9'
s
由大写英文字母、数字、和':'
组成
解题思路
提取范围信息:
- 使用
s.charCodeAt(0)
和s.charCodeAt(3)
提取范围的起始列和终止列对应的 ASCII 值。 - 使用
s[1]
和s[4]
提取范围的起始行和终止行的数字。
- 使用
遍历范围:
- 使用两层嵌套循环,外层遍历列范围,内层遍历行范围。
- 使用
String.fromCharCode
将列的 ASCII 值转换为字母。
构建单元格名称:
- 将列和行拼接成单元格名称,并存入结果数组。
返回结果数组。
复杂度分析
- 时间复杂度:
O(colCount × rowCount)
,两层循环,生成结果数组。 - 空间复杂度:
O(colCount × rowCount)
,结果数组占用的空间。
代码
/**
* @param {string} s
* @return {string[]}
*/
var cellsInRange = function (s) {
let res = [];
// 外层循环:遍历列范围,从起始列到终止列
for (let i = s.charCodeAt(0); i <= s.charCodeAt(3); i++) {
// 内层循环:遍历行范围,从起始行到终止行
for (let j = Number(s[1]); j <= Number(s[4]); j++) {
// 生成单元格名称并加入结果数组
res.push(String.fromCharCode(i) + j);
}
}
return res;
};
相关题目
题号 | 标题 | 题解 | 标签 | 难度 | 力扣 |
---|---|---|---|---|---|
168 | Excel 表列名称 | [✓] | 数学 字符串 | 🟢 | 🀄️ 🔗 |
171 | Excel 表列序号 | [✓] | 数学 字符串 | 🟢 | 🀄️ 🔗 |
1030 | 距离顺序排列矩阵单元格 | [✓] | 几何 数组 数学 2+ | 🟢 | 🀄️ 🔗 |