559. Maximum Depth of N-ary Tree
559. Maximum Depth of N-ary Tree
🟢 🔖 树
深度优先搜索
广度优先搜索
🔗 LeetCode
题目
Given a n-ary tree, find its maximum depth.
The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.
Nary-Tree input serialization is represented in their level order traversal, each group of children is separated by the null value (See examples).
Example 1:
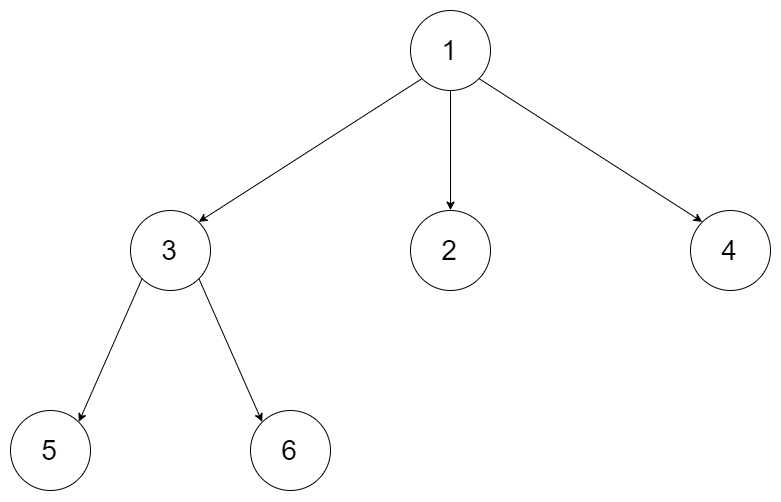
Input: root = [1,null,3,2,4,null,5,6]
Output: 3
Example 2:
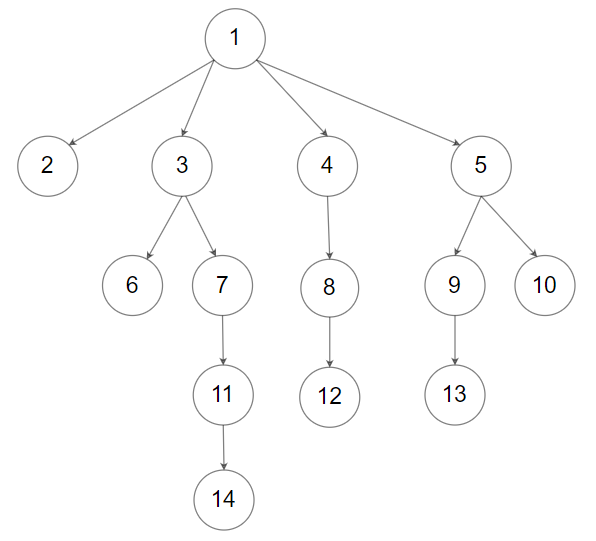
Input: root = [1,null,2,3,4,5,null,null,6,7,null,8,null,9,10,null,null,11,null,12,null,13,null,null,14]
Output: 5
Constraints:
- The total number of nodes is in the range
[0, 104]
. - The depth of the n-ary tree is less than or equal to
1000
.
题目大意
思路和 第 104 题 二叉树的最大深度 一样。
思路一:递归
只需求出根节点的每个子树的最大高度,取出其中的最大值再加一即为根节点的最大高度。
思路二:回溯
深度优先搜索(DFS)一颗 N 叉树,在 DFS 中,递归返回的时候,我们需要进行回溯(backtrack)。depth
变量用来记录当前节点的深度,每次进入一个子节点时,depth
增加,每次返回到父节点时,depth
需要减少。
代码
递归
/**
* @param {Node|null} root
* @return {number}
*/
var maxDepth = function (root) {
if (!root) return 0;
let max = 0;
for (let i of root.children) {
max = Math.max(max, maxDepth(i));
}
return max + 1;
};
回溯
/**
* @param {Node|null} root
* @return {number}
*/
var maxDepth = function (root) {
let depth = 0;
let res = 0;
const traverse = (root) => {
if (!root) return null;
depth++;
res = Math.max(depth, res);
for (let i of root.children) {
traverse(i);
}
depth--;
};
traverse(root);
return res;
};
相关题目
相关题目
- [104. 二叉树的最大深度](./0104.md)
- [2039. 网络空闲的时刻](https://leetcode.com/problems/the-time-when-the-network-becomes-idle)