257. Binary Tree Paths
257. Binary Tree Paths
🟢 🔖 树
深度优先搜索
字符串
回溯
二叉树
🔗 LeetCode
题目
Given the root
of a binary tree, return all root-to-leaf paths in any order.
A leaf is a node with no children.
Example 1:
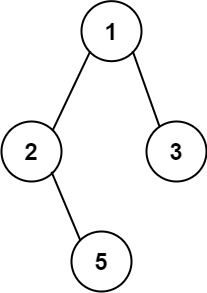
Input: root = [1,2,3,null,5]
Output: ["1->2->5","1->3"]
Example 2:
Input: root = [1]
Output: ["1"]
Constraints:
- The number of nodes in the tree is in the range
[1, 100]
. -100 <= Node.val <= 100
题目大意
给定一个二叉树,返回所有从根节点到叶子节点的路径。说明: 叶子节点是指没有子节点的节点。
解题思路
Google 的面试题,考察递归
代码
/**
* @param {TreeNode} root
* @return {string[]}
*/
var binaryTreePaths = function (root) {
let res = [];
if (!root) return res;
if (!root.left && !root.right) return ['' + root.val];
let tempLeft = binaryTreePaths(root.left);
for (let i of tempLeft) {
res.push(root.val + '->' + i);
}
let tempRight = binaryTreePaths(root.right);
for (let i of tempRight) {
res.push(root.val + '->' + i);
}
return res;
};
相关题目
相关题目
- [113. 路径总和 II](./0113.md)
- [988. 从叶结点开始的最小字符串](https://leetcode.com/problems/smallest-string-starting-from-leaf)
- [2096. 从二叉树一个节点到另一个节点每一步的方向](https://leetcode.com/problems/step-by-step-directions-from-a-binary-tree-node-to-another)